本文以商品修改为例,记录springmvc的注解开发,包括mapper,service,controller,@RequestMapping,controller方法的返回值等
需求
操作流程:
- 1.进入商品查询列表页面
- 2.点击修改,进入商品修改页面,页面中显示了要修改的商品。要修改的商品从数据库查询,根据商品id(主键)查询商品信息
- 3.在商品修改页面,修改商品信息,修改后,点击提交
开发mapper
mapper:
- 根据id查询商品信息
- 根据id更新Items表的数据
不用开发了,使用逆向工程生成的代码。
开发service
在com.iot.learnssm.firstssm.service.ItemsService
中添加两个接口
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
|
ItemsCustom findItemsById(Integer id) throws Exception;
void updateItems(Integer id,ItemsCustom itemsCustom) throws Exception;
|
在com.iot.learnssm.firstssm.service.impl.ItemsServiceImpl
中实现接口,增加itemsMapper
属性
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| @Autowired private ItemsMapper itemsMapper;
public ItemsCustom findItemsById(Integer id) throws Exception { Items items = itemsMapper.selectByPrimaryKey(id); ItemsCustom itemsCustom = new ItemsCustom(); BeanUtils.copyProperties(items, itemsCustom);
return itemsCustom; }
public void updateItems(Integer id, ItemsCustom itemsCustom) throws Exception {
itemsCustom.setId(id); itemsMapper.updateByPrimaryKeyWithBLOBs(itemsCustom); }
|
开发controller
方法:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69
| @Controller
public class ItemsController {
@Autowired private ItemsService itemsService;
@RequestMapping("/queryItems") public ModelAndView queryItems() throws Exception{ List<ItemsCustom> itemsList = itemsService.findItemsList(null);
ModelAndView modelAndView = new ModelAndView(); modelAndView.addObject("itemsList",itemsList);
modelAndView.setViewName("items/itemsList");
return modelAndView; }
@RequestMapping("/editItems") public ModelAndView editItems()throws Exception {
ItemsCustom itemsCustom = itemsService.findItemsById(1);
ModelAndView modelAndView = new ModelAndView();
modelAndView.addObject("itemsCustom", itemsCustom);
modelAndView.setViewName("items/editItems");
return modelAndView; }
@RequestMapping("/editItemsSubmit") public ModelAndView editItemsSubmit(HttpServletRequest request, Integer id, ItemsCustom itemsCustom)throws Exception {
itemsService.updateItems(id, itemsCustom);
ModelAndView modelAndView = new ModelAndView(); modelAndView.setViewName("success"); return modelAndView; }
}
|
@RequestMapping
定义controller方法对应的url,进行处理器映射使用。
1 2 3 4 5 6
| @Controller
@RequestMapping("/items") public class ItemsController {
|
出于安全性考虑,对http的链接进行方法限制。
1 2 3 4 5
| @RequestMapping(value="/editItems",method={RequestMethod.POST, RequestMethod.GET}) public ModelAndView editItems()throws Exception {
|
如果限制请求为post方法,进行get请求,即将上面代码的注解改为@RequestMapping(value="/editItems",method={RequestMethod.POST})
报错,状态码405:
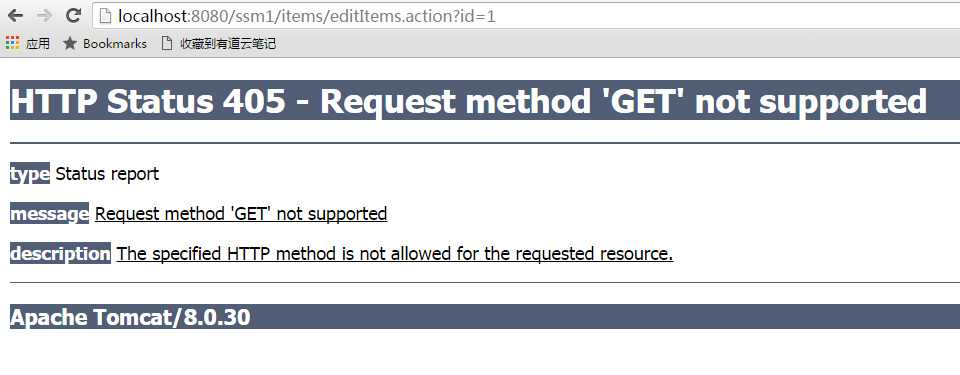
controller方法的返回值
需要方法结束时,定义ModelAndView,将model和view分别进行设置。
如果controller方法返回string
1.表示返回逻辑视图名。
真正视图(jsp路径)=前缀+逻辑视图名+后缀
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| @RequestMapping(value="/editItems",method={RequestMethod.POST,RequestMethod.GET})
public String editItems(Model model)throws Exception {
ItemsCustom itemsCustom = itemsService.findItemsById(1);
model.addAttribute("itemsCustom", itemsCustom);
return "items/editItems"; }
|
2.redirect重定向
商品修改提交后,重定向到商品查询列表。
redirect重定向特点:浏览器地址栏中的url会变化。修改提交的request数据无法传到重定向的地址。因为重定向后重新进行request(request无法共享)
3.forward页面转发
通过forward进行页面转发,浏览器地址栏url不变,request可以共享。
1 2
| return "forward:queryItems.action";
|
在controller方法形参上可以定义request和response,使用request或response指定响应结果:
1.使用request转向页面,如下:
request.getRequestDispatcher("页面路径").forward(request, response);
2.也可以通过response页面重定向:
response.sendRedirect("url")
3.也可以通过response指定响应结果,例如响应json数据如下:
1 2 3
| response.setCharacterEncoding("utf-8"); response.setContentType("application/json;charset=utf-8"); response.getWriter().write("json串");
|